So, I was messing around with Ruby the other day, trying to get a handle on how this whole “return” thing works. You know, it’s one of those things that seems simple enough on the surface, but then you start digging in, and it’s like, “Wait a minute, there’s more to this than meets the eye!”
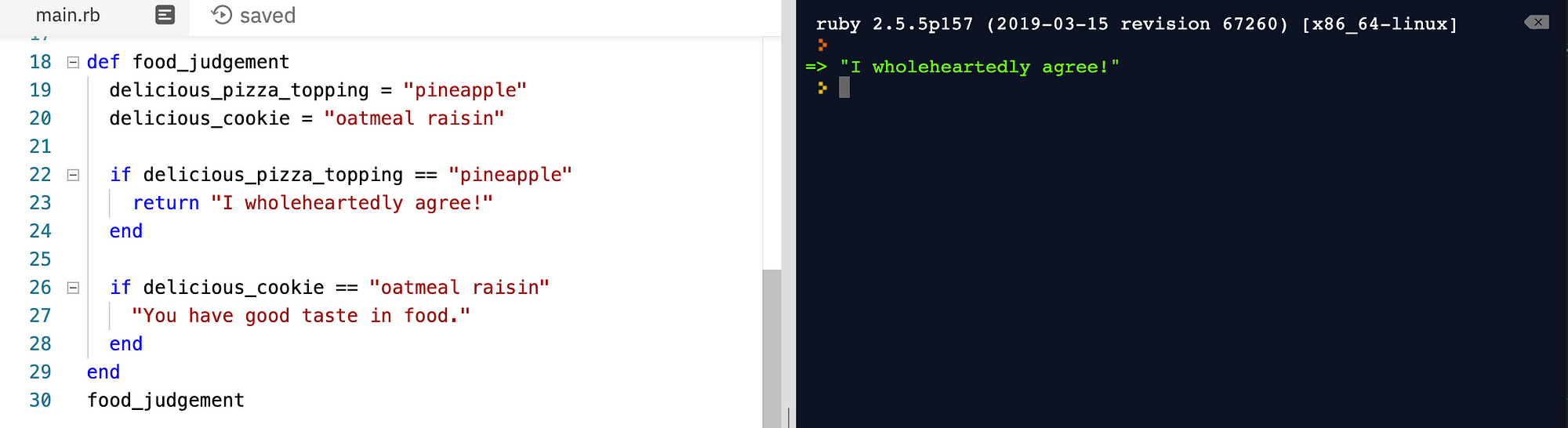
I started off by writing some basic methods, you know, just to see how it all played out. I mean, in Ruby, you don’t even need to explicitly use the “return” keyword most of the time. The last expression evaluated in a method is what gets returned automatically. It’s like Ruby’s saying, “I gotchu, I know what you mean.”
Here is a little code snippet I wrote:
-
def add(a, b)
-
a + b
-
end
See? No “return” in sight. But if you call `add(5, 3)`, you’ll still get 8. Pretty neat, huh? It is a simple method that adds two numbers. Ruby automatically returns the result of the last expression, which is a + b in this case.
But then I thought, “What if I do want to use ‘return’ explicitly? Does that change anything?” So I tried this:
-
def greet(name)
-
return “Hello, #{name}!”
-
“This will never be reached.”
-
end
I put a `return` statement right there in the middle of the method. And guess what? That last string, “This will never be reached,” it’s like it doesn’t even exist. When Ruby hits that `return`, it’s like, “Alright, I’m outta here!” It just exits the method right then and there, and whatever comes after is ignored. This method demonstrates an explicit return. When Ruby encounters the return keyword, it immediately exits the method and returns the specified value. In this case, it returns a greeting. The code after the return statement is never executed.
So, I played around with it some more, putting `return` statements in different places, inside loops, inside conditional blocks, you name it. And every time, it was the same deal. `return` means “That’s all, folks! We’re done here.”
I also found out that if you use `return` without any value after it, it just returns `nil`. It is like saying, “I’m done, and I have nothing to give back.” So here is a method that always returns nil, no matter what arguments you pass to it, because of the explicit return with no value.
-
def always_nil
-
return
-
end
And that’s pretty much my journey into the world of Ruby returns. It is a small but important part of the language, and understanding how it works can definitely help you write better, more predictable code. It is cool to see how such a simple keyword can have such a big impact on how your methods behave.
I still consider myself a beginner in Ruby, and sharing these experiences helps me solidify my understanding. I hope this little exploration of “return” was helpful to some of you out there. Keep coding and keep learning!